
Javascript class constructor code#
It is also possible that you might inherit code that contains such a bug. Hopefully, this is a mistake that you will catch quickly, but it can happen. In Example # 3, we have an example of a scenario that you don’t want: executing a JavaScript constructor function instead of instantiating it. HERE IS THE JS-FIDDLE.NET LINK FOR EXAMPLE # 1: Example # 2Ĭonsole. When we instantiate Foo, the variable “bar” becomes an instance of Foo, so bar.getMusic() returns “jazz”. Since the “music” property of Foo is: “jazz”, then the getMethod returns “jazz”. So, in Example # 1, the getMusic method returns “this.music”.
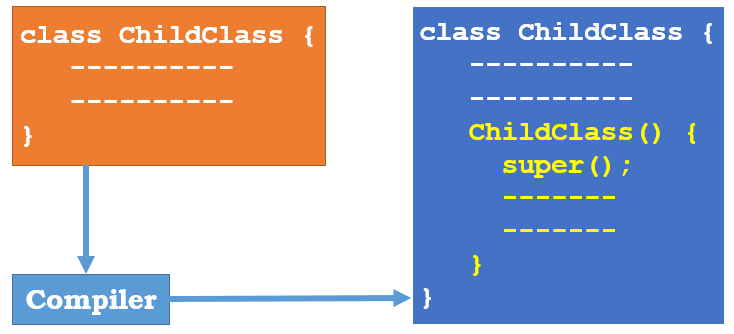
In other words, when you create your constructor function, you can use the “this” keyword to reference the object that WILL be created when the constructor is instantiated. The JavaScript “this” keyword has a special meaning inside of that object: it refers to itself. When you instantiate a JavaScript constructor function, an object is returned. But we don’t simply execute Foo we instantiate it: var bar = new Foo(). If we were to simply execute Foo as if it were a normal function, this would be true (and we will discuss this scenario in Example # 3). When you look at the code, it seems as if “this” will refer to the window object. If you truly want to understand constructor functions, it is important to remember how the JavaScript “this” keyword differs inside that constructor. The “instances” are JavaScript objects, but they differ from object literals in a few ways.įor an in-depth discussion of the difference between an object literal and an instance object, see the article: “What is the difference between an Object Literal and an Instance Object in JavaScript? | Kevin Chisholm – Blog”.Įarlier on, we established that inside a function that is not a method, the JavaScript “this” keyword refers to the window object. If you remember from previous articles, constructor functions act like classes, allowing you to define a “blueprint” object, and then create “instances” of that “class”.
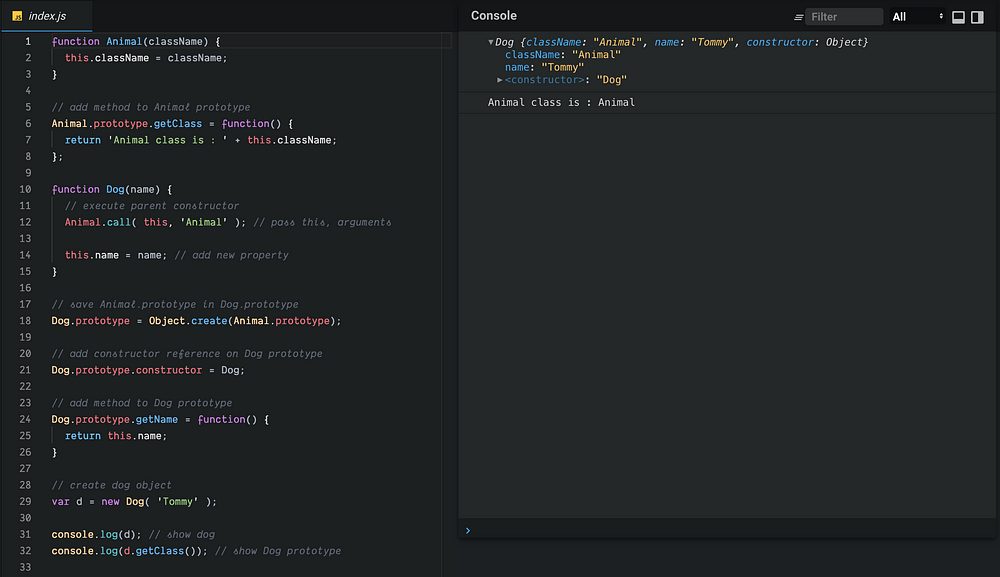
When you instantiate a JavaScript constructor function, the JavaScript “this” keyword refers to the instance of the constructor. In other words, the variable “bar” becomes an instance of “Foo”.

When we instantiate Foo, we assign that instantiation to the variable: “bar”. We’ve also created a constructor function named “Foo”. Using a Static Method Exampleīelow is an example for using a static method in JavaScript.When you instantiate a JavaScript constructor function, the JavaScript “this” keyword refers to the instance of the constructor. The important thing to note from the example above is that every static method begins with the static keyword. Creating Class Methods Exampleīelow is an example for creating class methods in JavaScript. A good method to add to the student class is one that generates a report on each student. This variable is important because it allows you to use the object that is created.Ī method is a function of a class that is used to perform operations on objects that are created from the class. Now you have a new student with the first name John, the last name Brown, and a start date of 2018. When creating an object of a class, you need to use the new keyword, followed by the class name and the values that you want to assign to the respective attributes. The code above uses the Student class to create an object. create a new objectĬonst john = new Student('John', 'Brown', ' 2018')
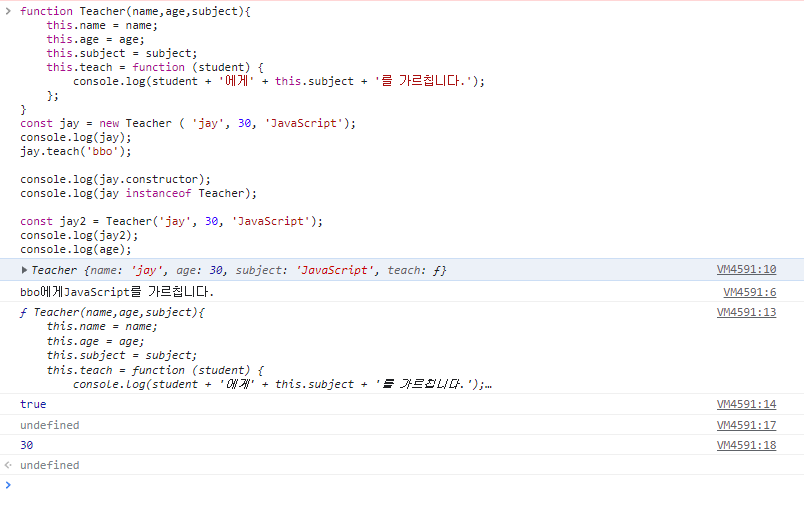
Creating an Object Exampleīelow, you'll see an example for creating an object in JavaScript. Looking at the student class constructor above, you can discern that an object of this class will have three attributes.
Javascript class constructor how to#
Related: How to Declare Variables in JavaScript However, this is not the case with JavaScript. Some languages will require that an attribute (variable) be declared before it can be used in a constructor or any other methods. Constructors are important in a language like JavaScript because they signify the number of attributes an object of a specific class should have.
